While a program is running, its data must be stored in a memory. How much memory is required for an item, and where and how it is stored, depends on it type
A running program uses two regions of memory to store data. They are usually known as stack and heap.
Stack
System takes cares of all stack manipulation. As a programmer you need to worry about stack explicitly
But understanding stack will give a better hand on the way of programming. So it will help you to understand C# literature.. ( oops what a name ‘C# literature’.. ;) )
Stack is an array of memory that acts as a last-in, first-out (LIFO) data structure. Usually we store several kinds of data
· The values of certain types of variables
· The program’s current execution environment
· Parameters passed to methods
- Additional Facts
· Data can be added to and deleted from the top of the stack
· Placing a data item at the top of the stack is called pushing item to the stack
· Deleting an item from the top of the stack is called popping the item from the stack
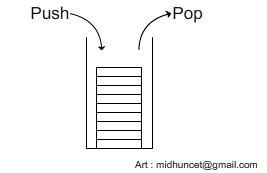
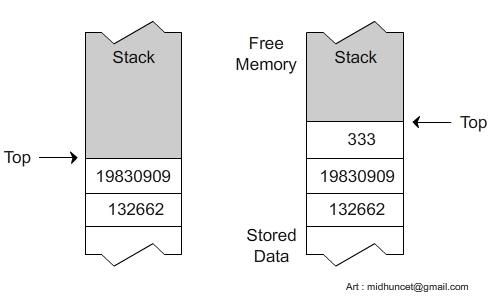
Heap
Heap is an area where chunks of memory can be allocated to store certain kinds of data.
No it’s not similar to Stack…
Unlike Stack, memory can be allocated and deallocated from heap in any order.
Theoretically we can say like that. Although our program can allocate memory from the heap, it cannot deallocate it. Instead the CLR’s Garbage Collector (GC) automatically cleans up orphaned heap objects when it determines that your code will no longer access them. This frees us from what in other programming languages can be an error-prone task.
This program has three allocated memory objects in the heap.
Later, one of this memory object is no longer used by the program.
The Garbage Collector (in .NET framework) finds the orphaned memory object
After garbage collection, the object’s memory has been released back to the heap
well that gives an idea of what stack and heap is...